Important
You are browsing the documentation for version 4.1 of OroCommerce, OroCRM and OroPlatform, which is no longer maintained. Read version 5.1 (the latest LTS version) of the Oro documentation to get up-to-date information.
See our Release Process documentation for more information on the currently supported and upcoming releases.
Customize Datagrids¶
Most business application users have to deal with significant amounts of data on a daily basis. Thus, efficiently navigating through large data sets becomes a must-have requirement and OroCommerce is not an exception. The application users must be able to easily filter, sort, and search through thousands (or millions) of records, usually represented in the form of a datagrid on a page.
This topic uses existing OroCommerce datagrids for illustration. If you are not familiar with OroPlatform datagrids, you may find it helpful to check the articles on how to create a simple datagrid, and how to pass request parameters to your datagrid. The datagrid.yml configuration reference and the OroDataGridBundle documentation contain additional useful information.
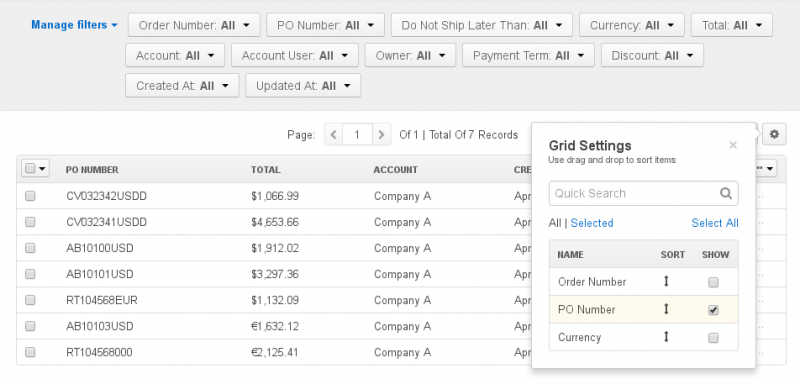
Data Sources¶
A datagrid is usually used to visualize some data coming from a data source. OroDataGridBundle allows for use of various data sources for datagrids, and includes the ORM data source adapter out of the box. It is possible to implement your own data source adapters as well.
The ORM data source types allow for database query specification, sorters and filters definitions to be specified in the datagrid configuration. Datagrid configuration can be supplied by a developer in YAML format. By convention, the datagrid.yml files placed in Resources/config folders of any application bundle are processed automatically. All supported data source configuration options that can be used in data source configuration section are described in the datasources section of the DataGridBundle documentation.
Inner Workings of Datagrids¶
Datagrids in Oro applications are highly customizable. It is possible to modify an existing grid in order to fetch more data than was originally defined in the grid configuration. In this article, we will retrieve and present to a user some additional data in the existing products-grid.
And before we start customizing it, let’s take a deeper look into two aspects of how the datagrids actually work:
Building and configuring a new DataGrid instance
Fetching data
Building Grids¶
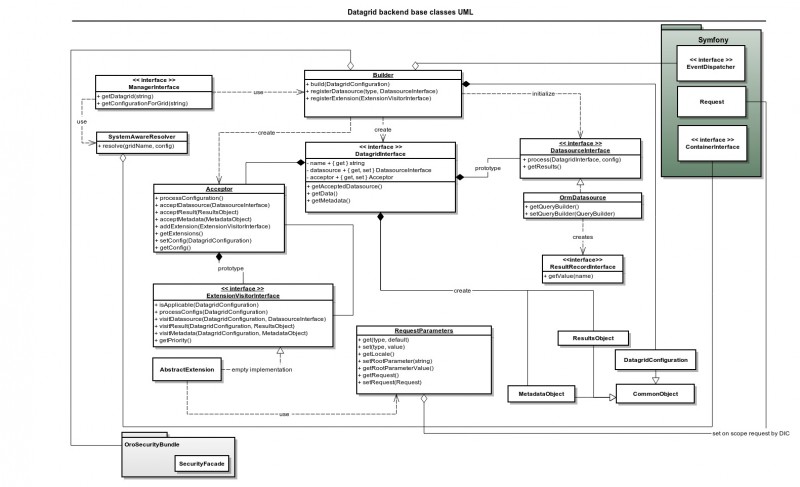
Datagrid\Builder class is responsible for creating and configuring an object and its data source. This is how the build method is processing the grid configuration internally:
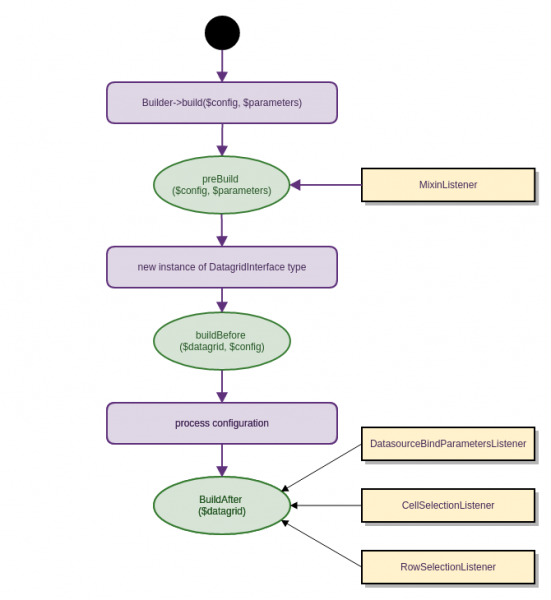
Imagine that you need to show a list of related price lists for every product record in the product grid. You want it to be displayed in a separate column with a multi-select filter. Also you want to add one more column to display owner of each of the product records.
One of the possible ways to customize this grid would be through events triggered by the system during the grid build process and when the data is fetched from the data source.
There are several events triggered before processing the datagrid configuration files. In this case, a good choice is the onBuildBefore event. By listening to this event you can add new elements to the grid configuration or modify already existing configuration in your event listener.
Note
More information about grid column definition configuration options is available in the columns and properties section of the DataGrid documentation.
The Product entity has a many-to-one relation with the Business Unit entity, so in order to add the owner column to the grid and load the owner data from the data source, you should modify its query configuration by adding additional join and select parts.
Fetching Data¶
However, the retrieving data for the price lists column is a little bit more complicated, because the Product entity has many-to-many relation with price lists, and the join result will contain duplicate rows. In such situations or when some other dynamic data should be included into the query results, a possible solution would be data modification after the rows were fetched from the data source.
This is how the data retrieval works in general:
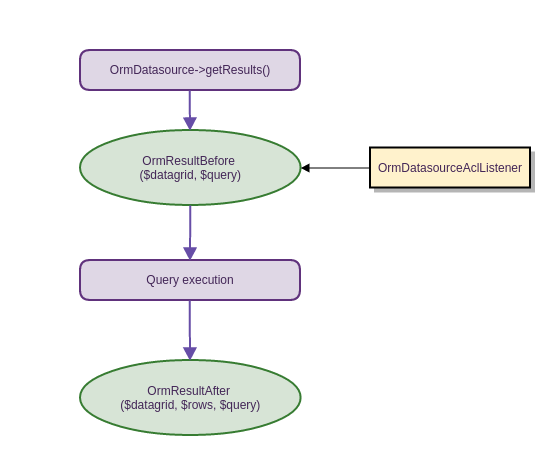
So in our customization, we will fetch the price list data in a separate query, and then we will attach this data to each of the product records in the grid.
Product Grid Customization¶
The resulting implementation of the ProductsGridListener may look similar to this example:
1class ProductsGridListener
2{
3 ...
4
5 /**
6 * @param BuildBefore $event
7 */
8 public function onBuildBefore(BuildBefore $event)
9 {
10 $datagridConfiguration = $event->getConfig();
11 $this->addBusinessUnitColumn($datagridConfiguration);
12 $this->addPriceListsColumn($datagridConfiguration);
13 }
14
15 /**
16 * @param OrmResultAfter $event
17 */
18 public function onResultAfter(OrmResultAfter $event)
19 {
20 $records = $event->getRecords();
21 $this->addPriceListsToRecords($records);
22 }
23
24 /**
25 * @param DatagridConfiguration $datagridConfiguration
26 */
27 protected function addPriceListsColumn(DatagridConfiguration $datagridConfiguration)
28 {
29 $column = [
30 'label' => 'Price Lists',
31 'type' => 'twig',
32 'template' => 'OroB2BPricingBundle:Datagrid:Column/price_lists.html.twig',
33 'frontend_type' => 'html',
34 'renderable' => true,
35 ];
36 $datagridConfiguration->addColumn('price_lists', $column);
37 }
38
39 /**
40 * @param DatagridConfiguration $datagridConfiguration
41 */
42 protected function addBusinessUnitColumn(DatagridConfiguration $datagridConfiguration)
43 {
44 $datagridConfiguration->joinTable(
45 'left',
46 [
47 'join' => BusinessUnit::class,
48 'alias' => 'business_unit',
49 'conditionType' => 'WITH',
50 'condition' => 'product.owner = business_unit',
51 ]
52 );
53
54 $column = [
55 'label' => 'Owner'
56 ];
57
58 // column name should be ther same as the field alias in the select query
59 $datagridConfiguration->addColumn('owner', $column, 'business_unit.name as owner');
60 }
61
62 /**
63 * @param ResultRecord[] $records
64 * @throws \Doctrine\ORM\ORMException
65 */
66 protected function addPriceListsToRecords(array $records)
67 {
68 $repository = $this->registry->getRepository(PriceListToProduct::class);
69 /** @var EntityManager $objectManager */
70 $objectManager = $this->registry->getManager();
71
72 $products = [];
73 foreach ($records as $record) {
74 $products[] = $objectManager->getReference(Product::class, $record->getValue('id'));
75 }
76
77 $priceLists = [];
78 foreach ($repository->findBy(['product' => $products]) as $item) {
79 $priceLists[$item->getProduct()->getId()][] = $item->getPriceList();
80 }
81
82 /** @var ResultRecord $record */
83 foreach ($records as $record) {
84 $id = $record->getValue('id');
85 $products[] = $objectManager->getReference(Product::class, $id);
86
87 $record->addData(['price_lists' => $priceLists[$id]]);
88 }
89 }
90}
We will need to register this event listener in the service container:
1grid_event_listener.product:
2 class: Oro\Bundle\CustomGridBundle\Datagrid\ProductsGridListener
3 arguments:
4 - '@doctrine'
5 tags:
6 - { name: kernel.event_listener, event: oro_datagrid.datagrid.build.before.products-grid, method: onBuildBefore }
7 - { name: kernel.event_listener, event: oro_datagrid.orm_datasource.result.after.products-grid, method: onResultAfter }
After the application cache is refreshed (or immediately in the dev mode) two new columns will appear in the product grid.
Custom Filters¶
Our second customization task will be to add filters for the newly introduced column.
In most cases, the built-in filters would work just perfectly. But in the case of the price lists column, a custom filter is required. The purpose of this filter will be to modify the data retrieval query depending on the filter values entered by a user.
1class ProductPriceListsFilter extends EntityFilter
2{
3 /**
4 * @var RegistryInterface
5 */
6 protected $registry;
7
8 /**
9 * @inheritdoc
10 */
11 public function apply(FilterDatasourceAdapterInterface $ds, $data)
12 {
13 /** @var array $data */
14 $data = $this->parseData($data);
15 if (!$data) {
16 return false;
17 }
18
19 $this->restrictByPriceList($ds, $data['value']);
20
21 return true;
22 }
23
24 /**
25 * @param RegistryInterface $registry
26 */
27 public function setRegistry(RegistryInterface $registry)
28 {
29 $this->registry = $registry;
30 }
31
32 /**
33 * @param OrmFilterDatasourceAdapter|FilterDatasourceAdapterInterface $ds
34 * @param array $priceLists
35 */
36 public function restrictByPriceList($ds, array $priceLists)
37 {
38 $queryBuilder = $ds->getQueryBuilder();
39 $parentAlias = $queryBuilder->getRootAliases()[0];
40 $parameterName = $ds->generateParameterName('price_lists');
41
42 $repository = $this->registry->getRepository(PriceListToProduct::class);
43 $subQueryBuilder = $repository->createQueryBuilder('relation');
44 $subQueryBuilder->where(
45 $subQueryBuilder->expr()->andX(
46 $subQueryBuilder->expr()->eq('relation.product', $parentAlias),
47 $subQueryBuilder->expr()->in('relation.priceList', ":$parameterName")
48 )
49 );
50
51 $queryBuilder->andWhere($subQueryBuilder->expr()->exists($subQueryBuilder->getQuery()->getDQL()));
52 $queryBuilder->setParameter($parameterName, $priceLists);
53 }
54}
Our new filter should be registered in the service container with the oro_filter.extension.orm_filter.filter tag:
1grid_filter.price_lists:
2 class: Oro\Bundle\CustomGridBundle\Filter\ProductPriceListsFilter
3 public: false
4 arguments:
5 - '@form.factory'
6 - '@oro_filter.filter_utility'
7 calls:
8 - [setRegistry, ['@doctrine']]
9 tags:
10 - { name: oro_filter.extension.orm_filter.filter, type: product-price-lists }
This filter can be added to the grid configuration similarly to how we added new columns – in an event listener. Thus the final implementation of the ProductsGridListener would look like this:
1class ProductsGridListener
2{
3 /**
4 * @var RegistryInterface
5 */
6 protected $registry;
7
8 /**
9 * @param RegistryInterface $registry
10 */
11 public function __construct(RegistryInterface $registry)
12 {
13 $this->registry = $registry;
14 }
15
16 /**
17 * @param BuildBefore $event
18 */
19 public function onBuildBefore(BuildBefore $event)
20 {
21 $datagridConfiguration = $event->getConfig();
22 $this->addBusinessUnitColumn($datagridConfiguration);
23 $this->addPriceListsColumn($datagridConfiguration);
24 $this->addPriceListsFilter($datagridConfiguration);
25 }
26
27 /**
28 * @param OrmResultAfter $event
29 */
30 public function onResultAfter(OrmResultAfter $event)
31 {
32 $records = $event->getRecords();
33 $this->addPriceListsToRecords($records);
34 }
35
36 /**
37 * @param DatagridConfiguration $datagridConfiguration
38 */
39 protected function addPriceListsColumn(DatagridConfiguration $datagridConfiguration)
40 {
41 $column = [
42 'label' => 'Price Lists',
43 'type' => 'twig',
44 'template' => 'OroCustomGridBundle:Datagrid:Column/price_lists.html.twig',
45 'frontend_type' => 'html',
46 'renderable' => true,
47 ];
48 $datagridConfiguration->addColumn('price_lists', $column);
49 }
50
51 /**
52 * @param DatagridConfiguration $datagridConfiguration
53 */
54 protected function addBusinessUnitColumn(DatagridConfiguration $datagridConfiguration)
55 {
56 $datagridConfiguration->joinTable(
57 'left',
58 [
59 'join' => BusinessUnit::class,
60 'alias' => 'business_unit',
61 'conditionType' => 'WITH',
62 'condition' => 'product.owner = business_unit',
63 ]
64 );
65
66 $column = [
67 'label' => 'Owner'
68 ];
69
70 // column name should be ther same as the field alias in the select query
71 $datagridConfiguration->addColumn('owner', $column, 'business_unit.name as owner');
72 }
73
74 /**
75 * @param DatagridConfiguration $datagridConfiguration
76 */
77 protected function addPriceListsFilter(DatagridConfiguration $datagridConfiguration)
78 {
79 $filter = [
80 'type' => 'product-price-lists',
81 'data_name' => 'price_lists',
82 'options' => [
83 'field_type' => 'entity',
84 'field_options' => [
85 'class' => PriceList::class,
86 'property' => 'name',
87 'multiple' => true
88 ]
89 ]
90 ];
91
92 $datagridConfiguration->addFilter('price_lists', $filter);
93 }
94
95 /**
96 * @param ResultRecord[] $records
97 * @throws \Doctrine\ORM\ORMException
98 */
99 protected function addPriceListsToRecords(array $records)
100 {
101 $repository = $this->registry->getRepository(PriceListToProduct::class);
102 /** @var EntityManager $objectManager */
103 $objectManager = $this->registry->getManager();
104
105 $products = [];
106 foreach ($records as $record) {
107 $products[] = $objectManager->getReference(Product::class, $record->getValue('id'));
108 }
109
110 $priceLists = [];
111 foreach ($repository->findBy(['product' => $products]) as $item) {
112 $priceLists[$item->getProduct()->getId()][] = $item->getPriceList();
113 }
114
115 /** @var ResultRecord $record */
116 foreach ($records as $record) {
117 $id = $record->getValue('id');
118 $products[] = $objectManager->getReference(Product::class, $id);
119
120 $record->addData(['price_lists' => $priceLists[$id]]);
121 }
122 }
123}
A fully working example, organized into a custom bundle, is available in the CustomGridBundle.zip file (Download 13.47 KB).
In order to add this bundle to your application, please extract the content of the zip archive into a source code directory that is recognized by your composer autoload settings.
Related Articles